Our SharePoint Admin was recently trying to dig up some details on exactly what permissions/functionality the
Manage Web Site permission provides when reviewing the permissions granted to a given permission level. The permission itself reads "Grants the ability to perform all administration tasks for the Web site as well
as manage content"; however, that doesn't provide the specific details that she was looking for. Just to be clear on what I'm talking about, you can access the permissions of a given permission level via the following steps:
- Log on to the root site of a site collection that you're designated as a site collection administrator of
- Click on Site Actions -> Site Permissions
- On the ribbon, locate the Manage section and click on Permission Levels
- Click on one of the links associated with any permission level
- Review the Permissions enumeration located on the right side of the screen
- Scroll down to the Site Permissions category
- Scroll down to the fourth checkbox and you will see the Manage Web Site permission
Unfortunately, I wasn't able to locate detailed information on what this permission provides so I decided to grind out an answer to her question by simply adding and removing the
Manage Web Site permission and comparing the difference between the capabilities that were listed under
Site Actions ->
Site Settings for a test account that was explicitly granted the permission level I was modifying. The results of this analysis are presented in the following screen shots:
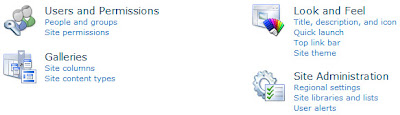 |
Image#1 - Site Settings without Manage Web Site |
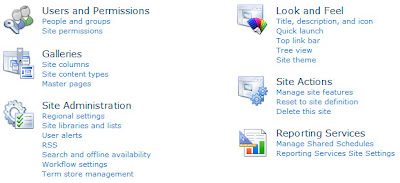 |
Image#2 - Site Settings with Manage Web Site |
Based on these results, the Manage Web Site permission provides the following capabilities:
- Look and Feel
- Tree view
- Ability to adjust whether the Quick Launch is enabled/disabled
- Ability to enable/disable a Tree View to aid navigation
- Galleries
- Master pages
- Access to the Master Page Gallery
- Site Administration
- RSS
- Ability to allow RSS feeds in the site
- Specify site-wide channel elements for the RSS feeds if enabled
- Copyright info
- Managing Editor
- Webmaster
- Time To Live (minutes)
- Search and offline availability
- Ability to choose whether or not the site appears in search results
- Specify the indexing behavior associated with site ASPX pages and content
- Ability to choose whether or not content can be downloaded to offline clients
- Workflow settings
- Ability to manage workflows on the site
- Term store management
- Ability to specify the Managed Metadata Service of the site as well as control who can manage the associated term store
- Site Actions
- Manage site features
- Ability to active/deactivate site features
- Reset to site definition
- Ability to restore the site back to its original site template
- Delete this site
- Ability to permanently delete the site
- Reporting Services
- Manage Shared Schedules
- Ability to manage schedule information associated with reports or subscriptions associated with the site
- Reporting Services Site Settings
- Ability to modify the default site settings for Reporting Services
- Enable client-side printing by allowing the RSClientPrint ActiveX control to be available for download from the site
- Enable local mode error messages to allow error messages to be passed to remote computers when running in local mode
- Enable accessibility metadata in the HTML output for reports
At this time, I haven't delved deeper into whether or not there are any differences within the functionality that is common between the two cases (i.e. both have the Quick Launch link active under Look and Feel); however, it's entirely possible there could be differences. For now, this research has provided us with enough information to satisfy our needs; therefore, I'm going to stop here for the moment. Hope this helps!